This WordPress plugin development tutorial teaches how to create a plugin for WordPress with a simple example of building a hello world plugin in WordPress.
In this short tutorial, I will show you how to create a custom WordPress plugin and print the “Hello world” text inside its admin page. This article also contains ten helpful tips for beginner WordPress developers.
If you do not know this already, WordPress plugins are stored inside the wp-content/plugins
folder, which can be accessed from your WordPress root directory.
In 2023 there will be around 60,500 plugins available to download from WordPress’s official plugins directory.
In this simple tutorial, we want to create a simple WordPress plug-in and put it inside the plugins folder, then activate it from the WordPress admin panel (wp-admin).
Creating a simple “Hello World” plugin in WordPress can be done in 3 easy steps:
- creating the plugin’s main file
- writing plugin headers in the created file (headers: information about the plugin and the author)
- writing functions to display “Hello World” text inside an admin page in the WordPress panel
So here we go:
1- Create your hello world plugin main file
The plugin’s main file will contain all of our headers and functions that will be discovered by WordPress automatically. this file must be inside a folder with the same name in the wp-content/plugins
directory.
in this tutorial, I’m going to call this plugin “Hello World”, so go ahead and:
-> create a “hello-world” folder inside the wp-content/plugins
directory of your WordPress installation (using FTP or file manager).
-> create a file named hello-world.php inside this hello-world folder that you just created.
2- Write plugin headers for this hello world plugin
headers are information about the module (plugin). without the headers, WordPress can NOT discover your plugin.
Plugin Name is required in plugin headers, and other headers are optional.
This means that you can only set a name for a plugin and activate it, but you can also write complete information about this plugin to be displayed on the plugins page inside the admin panel of WordPress.
You can write your information as the author of the plugin inside headers for others to see if they’ve installed your plugin.
This is the full code of a proper header for the WordPress hello world plugin. You can edit this information based on yours.
-> these codes will go to the top of your hello-world.php file:
<?php
/**
* Hello World
*
* @package HelloWorld
* @author Mehdi Nazari
* @copyright 2019 Mehdi Nazari
* @license GPL-2.0-or-later
*
* @wordpress-plugin
* Plugin Name: Hello World
* Plugin URI: https://mehdinazari.com/how-to-create-hello-world-plugin-for-wordpress
* Description: This plugin prints "Hello World" inside an admin page.
* Version: 1.0.0
* Author: Mehdi Nazari
* Author URI: https://mehdinazari.com
* Text Domain: hello-world
* License: GPL v2 or later
* License URI: http://www.gnu.org/licenses/gpl-2.0.txt
*/
Now you have a folder inside the plugin’s directory named “hello-world” inside that, you have a file named “hello-world.php“, and inside that file, you have the above code.
At this point, you can activate your plugin, and WordPress will be ok with it.
After login into the admin dashboard (wp-admin), you can navigate to the plugins page by clicking on its menu item.
Or replace your website name with this URL:http://sitename.com/wp-admin/plugins.php
Find the hello world plugin in the plugins list. You can activate the plugin by clicking on the “Activate” button under the plugin’s name:

to display the “Hello World” text, you must create an admin page and display this text inside it.
3- Create an admin page for the hello world plugin
if you create a menu page for the hello-world plugin, its menu item will be automatically created, and you can access it from the WordPress menu list.
-> to create an admin page and a menu item for the hello-world plugin, copy this code and paste it at the bottom of the “hello-world.php” file:
function display_hello_world_page() {
echo 'Hello World!
';
}
function hello_world_admin_menu() {
add_menu_page(
'Hello World',// page title
'Hello World',// menu title
'manage_options',// capability
'hello-world',// menu slug
'display_hello_world_page' // callback function
);
}
add_action('admin_menu', 'hello_world_admin_menu');
now you can see the Hello World menu item inside the admin menu list if you just refresh the admin page (no reactivation is required). like this:
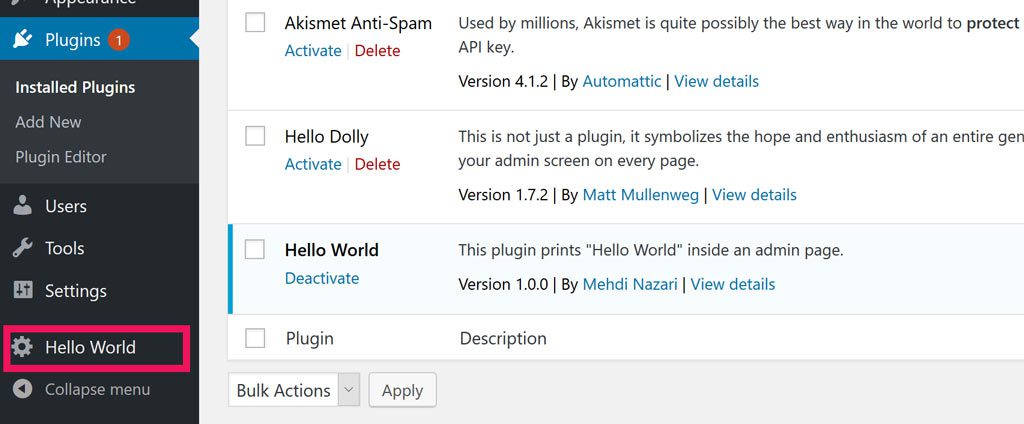
After clicking the “Hello World” menu item, you will navigate to its admin page, where you can see the “Hello World!” text that we printed using PHP’s echo command. like this:
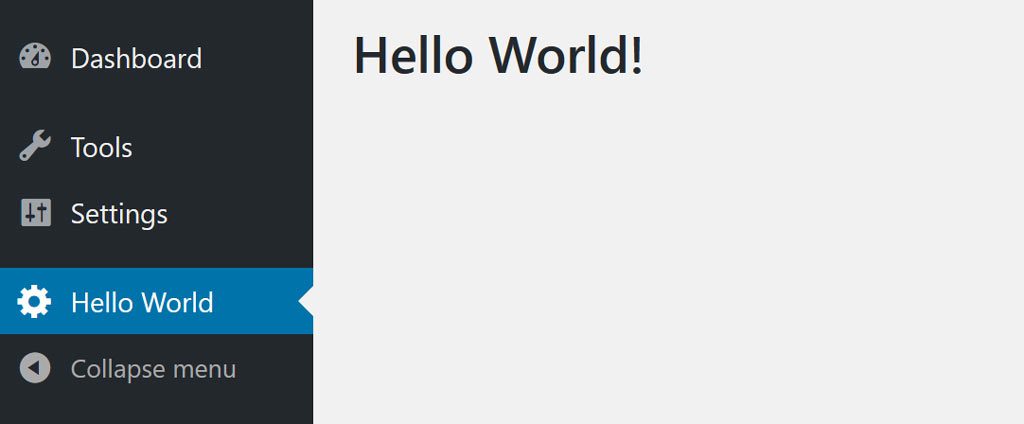
above codes explain:
First, we declared a function named display_hello_world_page()
to display the “Hello World!” text using the PHP’s echo command.
to make this function work as a page, we must register this function as the callback function of WordPress’s function add_menu_page()
. which is a WordPress core function used to create admin pages and menu links.
to execute the function add_menu_page()
, we must fire it using an action.
to create an action in WordPress, we use add_action()
function.
add_action()
function will take two parameters; the first parameter declares when the action is firing, while the second parameter declares what function must be fired.
add_action('admin_menu', 'hello_world_admin_menu')
is saying to WordPress that we want to run hello_world_admin_menu()
when admin_menu
action is running by WordPress.
With this add_action, our add_menu_page function will run when WordPress is trying to create admin menus.
add_menu_page()
is a WordPress core function that takes 7 parameters and creates an admin menu page for you (easy, right?).
These 7 parameters are “page title”, “menu title”, “capability”, “menu slug”, “callback function”, “icon url” and “position in menu”.
page title: this will be displayed on browser tabs.
menu title: this will be displayed as a menu name inside the WordPress panel.
capability: this one determines what kind of admin user can access this page.
menu slug: with this parameter, you can set an address for your admin page.
callback function: what function do you want to run when this page is called? we will print “Hello World!” in this function.
icon URL: if you want to use another icon other than the gear, you can write its URL here.
position in the menu: this is optional; if you want to position this item at a specific location in the WordPress menu, you can put the number here.
we did not use the last two parameters in our hello-world plugin and let it be the default.
you can read more about the add_menu_page
function in WordPress’s official documents.
Also, these tutorials can help you develop a perfect WordPress plugin:
- Learn how to activate a plugin automatically
- Learn how to get a plugins directory path
- Learn how to add a custom setting section
- Learn how to add a custom shortcode
- Learn how to get posts
- Learn how to get categories, tags (taxonomies)
- Learn how to extend WordPress posts query
If you want to develop flawless WordPress plugins, read these ten tips:
10 Things to remember when creating WordPress plugins as a beginner
if you are creating a WordPress plugin for the first time, you better keep a few things in mind. Here is the list:
1- You can include PHP files in your main plugin file
Like any other PHP script, you can include other PHP files in the main file. so you can include other PHP files inside your plugin’s main file (in our case hello-world.php file)
You can categorize and put your functions in different files to keep your codes clean and simple. You have to include them in your main file.
for example, you can split our hello-world.php file into two files like this:
-> create a file named functions.php next to your hello-world.php file.
Add these codes to this new functions.php file:
<?php
add_action('admin_menu', 'hello_world_admin_menu');
function hello_world_admin_menu() {
add_menu_page(
'Hello World',// page title
'Hello World',// menu title
'manage_options',// capability
'hello-world',// menu slug
'display_hello_world_page' // callback function
);
}
function display_hello_world_page() {
echo 'Hello World!
';
}
-> change your hello-world.php file’s content to this:
<?php
/**
* Hello World
*
* @package HelloWorld
* @author Mehdi Nazari
* @copyright 2019 Mehdi Nazari
* @license GPL-2.0-or-later
*
* @wordpress-plugin
* Plugin Name: Hello World
* Plugin URI: https://mehdinazari.com/how-to-create-hello-world-plugin-for-wordpress
* Description: This plugin prints "Hello World" inside an admin page.
* Version: 1.0.0
* Author: Mehdi Nazari
* Author URI: https://mehdinazari.com
* Text Domain: hello-world
* License: GPL v2 or later
* License URI: http://www.gnu.org/licenses/gpl-2.0.txt
*/
include_once("functions.php");
//or use include(),require(),require_once()
everything must stay the same after this change. we just made our codes a little more clear.
2- You can use the MVC approach in creating WordPress plugins
Yes, you can create WordPress plugins using the MVC approach.
You have two ways to do this. First, you can create your folder structure and classes.
Or you can use the WP-MVC plugin, which provides MVC folder structure and default classes for you, and you can extend them to achieve what you want.
WP-MVC will take care of file includes if you use it right, and it has helpful functions too.
Someday i will create a full guide for plugin development using WP-MVC.
3- Actions and filters are the primary way to connect with WordPress
Action and filter hooks are the most important part of the WordPress API. if you want to be a WordPress developer, you better know how they work.
An action hook will be triggered when an action happens. Like triggering a function when the user is just logged in (or failed).
Filters hook will be used to control WordPress core functions flow or change its functions output.
I created a full tutorial about WordPress action and filter hooks. read it here:
WordPress Action and Filter Hooks Tutorial (+10 Examples)
4- Not all functions need to be inside a plugin
You can also write your functions inside your template functions.php file.
If you do not have lots of code and functionalities in your plugin, you can write these codes inside function.php
file of our template. These functions will act the same as they are in a plugin.
Use action and filter hooks inside your template’s functions.php file.
functions.php file is located in wp-content/themes/yourtheme
directory.
By yourtheme, I mean your currently active theme folder.
5- Advanced WordPress plugin development can be a lot more complicated
If you want to create a plugin that can modify some functionality in WordPress, it will not take long and it will not be complicated in most cases.
But not all WordPress plugins are easy to create; sometimes, it will take months to create a WordPress plugin that works and has no bugs or issues.
Personally, I worked sometimes for a few months on a single plugin.
It depends on how much WordPress functions can help you achieve what you want. Sometimes you must write thousands of lines of code to achieve your needs.
To speed up your plugin development process, you better use assistant tools for developers.
6- You must always be concerned about the security of your WordPress plugin
When it comes to security, you should always think twice.
The main difference between an expert and a beginner developer is that the expert will always code securely and check the security of his scripts.
Some of the best practices for WordPress plugin development security for beginners:
- sanitize and validate user input values
- use “wp nonce” to prevent CSRF attacks on forms
- check file extensions in upload forms
- log important actions done by users
- use secure external PHP libraries
- check admin privileges on your custom functions (use user_can() function)
7- Keep your WordPress plugin files and codes nice and simple
After a few days of developing a WordPress plugin, codes can get messy and hard to understand, especially if your code is only in one file.
The best practice for coding clean is to separate different functions based on usage.
For example, put all your mathematical functions in a file named math_helper.php
and include it where you want to use it.
Using the MVC approach is the best way to keep codes clean and functions separated. That’s why WP MVC is awesome.
By default, WordPress is not MVC. But you can code in MVC if you want. You need to create folders and files for it.
WP MVC plugin already took care of folder structure, and file includes. You can try it for your next WordPress plugin development.
8- In WordPress plugin development, you can save data in a Database easily, but be careful
WordPress provides a global object named $wpdb
, you can always call this object and use it by writing global $wpdb;
code in your function. Something like this:
<?php
function insertDataToDatabase(){
global $wpdb;
$table_name = $wpdb->prefix . "custom_table";
$wpdb->insert($table_name, array('name' => "Test", 'email' => "test@test.com") );
}
$wpdb
object contains all of the database functions that you need.
in the above code, we used insert()
function of $wpdb
object to insert our data to custom_table table in the database.
You should be careful when working with a database, especially when saving user inputs.
Remember this: never trust the user’s input.
If you are inserting data with a direct query to the database, you must always sanitize the user’s input and validate its data type.
you can use sanitize_text_field()
function of WordPress to sanitize text fields; also, WordPress provides a sanitize function for all data types. For instance, sanitize_email()
sanitizes the user’s email input.
9- Toxic functions and infinitive loops inside a WordPress plugin can break your website
like any other PHP script, toxic functions can break the whole system.
Like any other PHP script, toxic functions can break the whole system.
By using the term “toxic functions” I mean functions that use lots of resources and can cause recourse shortage for your hosting server.
Infinitive loops inside a WordPress plugin or theme can break the system easily and cause lots of trouble for the host server.
Infinitive loops are programming loops that will never end. Because of a lack of break conditions or wrong conditions, these loops will run until your server is out of resources like CPU power or RAM.
You better learn how to handle frequently used functions like query posts using get_posts in an efficient way.
10- You can use CSS, jQuery, and JavaScript to create stunning layouts for your WordPress plugins
there are 3 ways you can add custom CSS and JS codes to your plugin’s admin page.
- use inline CSS and JS codes
- including CSS and JS using
<style>
and<script>
tags on pages - including external CSS and JS using WordPress
wp_enqueue_style()
andadmin_enqueue_scripts()
functions.
After including your custom CSS and JS codes, you can use them to stylize your WordPress plugin’s page.
you can include CSS and JS frameworks like Bootstrap and create your layout based on them.
jQuery is enabled by default on the WordPress admin page. you can write jQuery codes and run them without including the jQuery library. (it’s already included)
written by Mehdi Nazari about in WordPress WordPress Plugin Development
What do you think about "How to Create a Hello World Plugin for WordPress [2023]"?