The absint function in WordPress accepts a number and converts it to a positive integer. This function also converts a float number to a positive integer value. But what is the return if you pass Boolean or Array instead? Find the answer in my 7 examples of using absint() in WordPress development.
absint
Function Introduction
The absint() function in WordPress accepts a number and returns the absolute value for that number. The number can be negative or positive, defined as an integer, float, or string.
There is also the possibility of passing an array, boolean or null to this function. In such cases, absint()
somehow manages to return a legitimate value in return (keep reading for the examples).
How does the absint
function work in WordPress?
Since WordPress V2.5.0, The absint function is declared in wp-includes/function.php
file, so it’s available everywhere when developing the front end or admin panel of WordPress.
This function uses the PHP abs()
function under the hood. But before passing the number to PHP abs()
, WordPress absint()
converts the given value to an integer.
Because of this conversion, there is no possibility of getting a float value in return. Only returning an Integer is expected from the absint()
function.
Another important thing to note is that only the integer part of a giving decimal is considered by absint()
. And it does NOT round your number to the nearest integer.
For example, -3.99 is treated the same as -3.33; both will be considered -3, the integer part of both decimals.
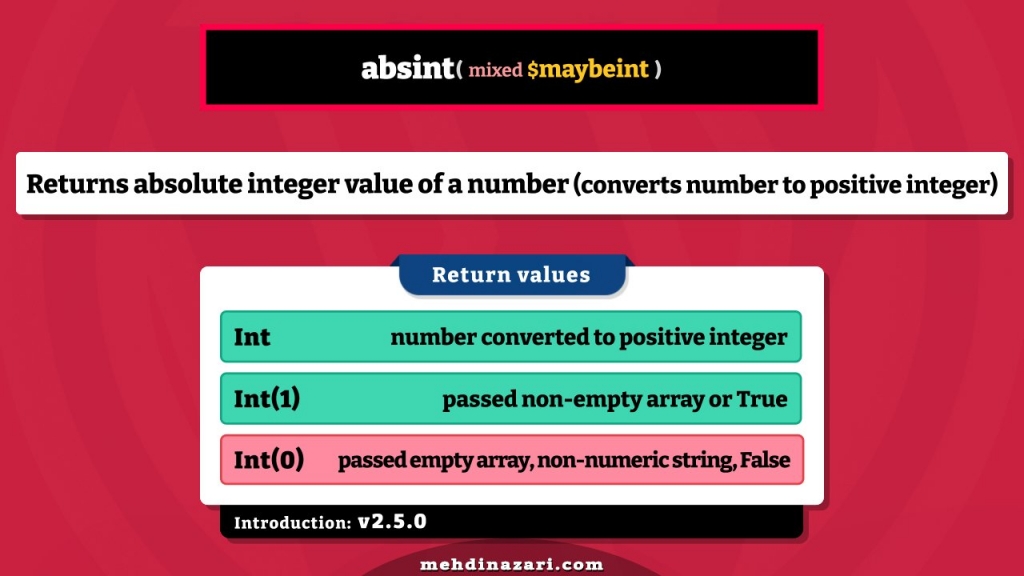
What is the absint
function use case in WordPress development?
If you have a number with the possibility of wrongly being declared negative, it’s wise to use absint()
to ensure it will be positive regardless.
But this function also returns legitimate values if you pass non-integers.
If you know the returning possibilities, you can use the absint()
function in other scenarios.
One good thing about using the absint
function is that the return value is always an integer.
Also, if your giving data type is String (containing only a number), you do not need to worry about converting it to an actual numerical type before passing it to the function.
absint
Function Syntax
The syntax is clear. absint()
only accepts one parameter, which can be an integer or not. So they’ve called it $maybeint
, because it may be an integer, or maybe not!
absint( mixed $maybeint )
absint
Function Parameter
$maybeint
parameter is required by the absint
function to calculate the returning value based on it.
You can pass a negative or positive integer, a negative or positive float, a string containing a number (or not), an array, a boolean, etc.
But maybe, in some cases, the absint()
does not return value you expect if you pass non-numerical data types.
Here are the possibilities of return value based on the passed data type:
absint
Function Result
If you pass one of the below data types to the absint
function, you’ll get:
- Integer:
- Positive integer -> the same passed number.
- Negative integer -> the passed number converted to positive.
- Float: integer part of the passed number (ex: 3 from 3.99), converted to positive if negative.
- String:
- Numerical only string -> the same as passing integer or float.
- Non-Numerical string -> 0.
- String starting with a number -> the starting number will be used as passed value.
- String with number in between characters -> 0.
- Math operation strings -> not supported, then 0.
- Array:
- Array with elements -> 1.
- Empty array -> 0.
- Boolean:
- True -> 1
- False -> 0
- Null: returns 0.
- Object: PHP warning with the text ‘Warning: Object of class …….. could not be converted to int’.
I tried passing all these data types to the WordPress absint
function and analyzing the return value in each case.
Continue reading for the result I got from each test (I have surprises for you!).
If you want to try the examples yourself, you better create a plugin and copy these PHP codes inside it. Check out my tutorial on how to create a hello world plugin for WordPress.
absint
Function Examples in WordPress Development
Here are seven examples (actually a lot more!) of using absint
function in WordPress development, with each data type:
Integer value for absint
function
In case of passing a positive integer to WordPress’ absint()
, You’ll get the same integer you passed as a return. But a negative integer will be converted to a positive in return.
So this way absint()
enforces integer numbers to be positive regardless of their original definition. Example:
//defining a negative integer
$negative = -5;
//defining a positive integer
$positive = 7;
//defining a zero integer
$zero = 0;
//defining a variable based on math operation
$multiply = $negative * $positive;
/*now we check above variables in absint():*/
//prints 5
echo absint($negative) .'<br>';
//prints 7
echo absint($positive) .'<br>';
//prints 0
echo absint($zero) .'<br>';
//prints 35
echo absint($multiply);
Float value for absint
function
absint()
function will convert the given float number to an integer and return it as a non-negative.
The below example is important. Especially because of unexpected behavior reported in absint by Joel James.
//defining a negative float
$negative = -5.99;
//defining a positive float
$positive = 7.99;
//defining a variable based on math operation
$multiply = $negative * $positive;
/*now we check above variables in absint():*/
//prints 5
//-5 is the integer part of -5.99, then it converts to positive
echo absint($negative) .'<br>';
//prints 7
//7 is the integer part of 7.99
echo absint($positive) .'<br>';
//prints 47
//-5.99 x 7.99 = -47.8601 -> -47 converts to 47
echo absint($multiply).'<br>';
//👇
//****unexpected behaviour****//
//reported by Joel James: https://developer.wordpress.org/reference/functions/absint/#comment-3310
//because of percision settings, below example returns 1998 instead of 1999
echo absint(-19.99 * 100);
//👇
//But if you increase float data percision, problem will be fixed (if it is a problem for you!)
//example below prints 1999
echo absint(-19.999 * 100);
One thing to remember is that there is no exact 1, 2, 3, 4, … in float numbers. Instead, there is 1.9999999999999, then it goes to 2.0000000000001 (the ‘2’ number is not in between).
Note that the precision needed to go to the next integer differs based on operating systems and PHP settings.
You can try this in below example (it’s fun :)):
//prints 1
echo (int) 1.999.'<br>';
//again prints 1
echo (int) 1.999999.'<br>';
//still prints 1
echo (int) 1.999999999.'<br>';
//now prints 2 (you may need different precision)
echo (int) 1.9999999999999999.'<br>';
//prints 1 again!
echo (int) 1.9999999999999998.'<br>';
String value for absint
function (This one can be surprising!)
If the string contains only a number, integer, or float, it will be treated the same as int or float.
But if you pass a character-based string without numbers, absint()
will return 0.
What if a number is presented in the string, mixed with non-numerical characters? well… it depends on the position of that number!
If a number is at the beginning of the passed string (ex: ‘-123 text text’), absint()
will consider the starting number as the passed number (in our example, 123 will be returned.
On the other hand, If a number is mixed in between the passed string (ex: ‘text -123 text’), absint()
will return 0. same result for a number appending at the end of the string (ex: ‘text text -123).
//defining a string value
$stringOnly = 'this is a test';
//defining a string value mixed with a number in between
$stringWithNumberInBetween = 'this 123 is a test';
//defining a string value mixed with a number at beginning
$stringWithNumberAtBeginning = '-123 is a test';
//defining a string value mixed with a number at the end
$stringWithNumberAtTheEnd = 'this is a 123';
//defining a string of negative integer
$negativeIntegerString = '-123';
//defining a string of positive integer
$positiveIntegerString = '123';
//defining a string of negative float
$negativeFloatingString = '-5.99';
//defining a string of positive float
$positiveFloatingString = '7.99';
//defining a string containing a math operation
$mathOperationString = '-5 * 7';
//defining a string containing a math operation with Parenthesis
$mathOperationStringWithParenthesis = '(-5 * 7)';
/*now we check above variables in absint():*/
//prints 0
echo absint($stringOnly) . '<br>';
//prints 0
echo absint($stringWithNumberInBetween) . '<br>';
//prints 123
echo absint($stringWithNumberAtBeginning) . '<br>';
//prints 0
echo absint($stringWithNumberAtTheEnd) . '<br>';
//prints 123
echo absint($negativeIntegerString) . '<br>';
//prints 123
echo absint($positiveIntegerString) . '<br>';
//prints 5
echo absint($negativeFloatingString) . '<br>';
//prints 7
echo absint($positiveFloatingString) . '<br>';
//prints 5 (you expected 35, didn't you? 😆)
//it seems that absint() does not handle math operations inside string!
echo absint($mathOperationString) . '<br>';
//prints 0 (still waiting for 35? 😂)
//absint() does not handle math operations inside string even with parenthesis (now its 0!)
echo absint($mathOperationStringWithParenthesis) . '<br>';
Array value for absint
function
If you pass an array with elements inside it, absint()
will return 1. But passing an empty array will get 0 in return. Example:
//defining an empty array
$emptyArray = array();
//defining an array containing numbers
//Note it does not matter! I just want to show you.
$arrayOfNumbers = array(-1, 0, 1, 2, 3);
//defining an array containing characters (not different anyway!)
$arrayOfStrings = array('test', 'another test');
/*now we check above variables in absint():*/
//prints 0
echo absint($emptyArray) .'<br>';
//prints 1
echo absint($arrayOfNumbers) .'<br>';
//prints 1
echo absint($arrayOfStrings);
Boolean value for absint
function
//prints 0
echo absint(false) . '<br>';
//prints 1
echo absint(true);
Null value for absint
function
//prints 0
echo absint(null);
Object value for absint
function
//defining the object to be tested if absint()
$object = new stdClass();
$object->test = -6.99;
//passing the object itself throws PHP warning:
//Warning: Object of class stdChjlass could not be converted to int in ...
echo absint($object);
//note that if you pass test field of $object to absint(), it will not throw warning
//it will be converted to positive integer (6 in this example)
echo absint($object->test);
written by Mehdi Nazari about in WordPress WordPress Functions
What do you think about "How to Use absint Function in WordPress [7 Examples]"?