Learn how to add a new section to the settings page of the WordPress admin panel with examples. In this WordPress tutorial, we'll introduce the WordPress add_settings_section function and review its usage with examples.
add_settings_section
Function Introduction
Since WordPress V2.7.0 The add_settings_section
Function is responsible for adding a new section to the settings page inside the WordPress admin panel.
To add a new section to the settings page, you need to give this function an ID to set on the section, a title to display for the admin, a callback function to print what you need in this section, and the slug of the settings page.
The last parameter in the add_settings_section is an optional array you can use to customize your settings section. This array was introduced in WordPress V.6.1.0.
Basic usage of add_settings_section function:
add_settings_section(
'id_for_settings_section',
'A custom title to show',
'my_custom_section_callback',
'general',
//since V6.1.0
array(
//prints before the section
'before_section' => '<div class="before-section">',
//prints after the section (does not print in empty section)
'after_section' => '</div>', //html for after the section
)
);
Note: please update WordPress to the latest version before using this function. Because there was a major change in the
add_settings_section()
since WordPress V.6.1.0.
add_settings_section
Function Syntax
Look at the syntax below; there are four required string parameters and an optional array accepted by the add_settings_section function:
add_settings_section( string $id, string $title, callable $callback, string $page, array $args )
add_settings_section
Function Parameters
$id
: ID string to set for this section. Use a hyphenated string (slug).$title
: this string is used to show a heading title for the new section.$callback
: to print the content of this section. (Not the form fields. They are added using theadd_settings_fields
function)$page
: default options are: general, reading, writing, discussion, and media. If you added a custom settings page, you can use its slug here to add a section. (add new page usingadd_options_page()
)$args
: This array was recently introduced (since WordPress V6.1.0) to add HTML codes before or after the section by these array keys:before_section
: HTML code to put before the section contentafter_section
: HTML code to put after the section content. (does not print for empty sections)
add_settings_section
Function Example
In this example, we’ll use add_settings_section with add_settings_field to print a section with sample fields.
//callback to print a simple section in general page
function my_custom_section_callback(){
echo 'This text is inside the callback!
';
}
//callback to print a simple input field
function my_custom_field_callback(){
echo '';
}
function add_my_custom_section_to_settings(){
//add the section to general page in admin panel
add_settings_section(
'id_for_settings_section',
'A custom title to show',
'my_custom_section_callback',
'general',
array(
'before_section' => 'Text Before the Section', //html for before the section
'after_section' => 'Text After the Section', //html for after the section
)
);
//add a sample field to this section.
add_settings_field(
'id_for_setting_field',
'A custom field',
'my_custom_field_callback',
'general',
//put the id of custom section here:
'id_for_settings_section'
);
}
add_action('admin_init', 'add_my_custom_section_to_settings');
After using this code in your WordPress custom plugin or functions.php of the active theme, You can open this URL to see the section:
http://siteurl.com/wp-admin/options-general.php
Look for something like this screenshot:
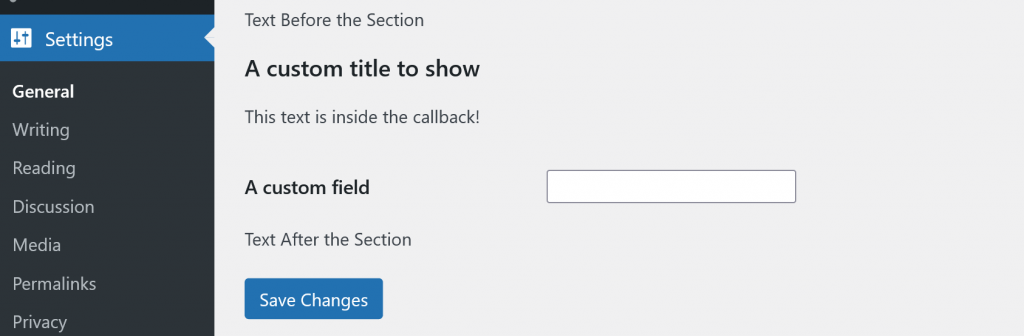
written by Mehdi Nazari about in WordPress WordPress Functions WordPress Plugin Development WordPress Theme Development
What do you think about "How to Add a Custom Section to Settings Page in WordPress"?